“Explore the Vue 3 Composition API in depth! Learn how to use the setup()
function, create components, and manage reactivity with ref()
and reactive()
. Includes examples, interview questions, and tips for scalable Vue.js applications.”
The Vue 3 Composition API is a powerful addition to Vue.js, offering developers greater flexibility and reusability in structuring their applications. This article explores the key concepts of the Composition API, focusing on its introduction, creating components using the setup()
function, and handling reactive references with ref()
and reactive()
.
Table of Contents
1. Introduction to the Composition API
The Composition API was introduced in Vue 3 to address limitations in the Options API, such as the difficulty of organizing and reusing logic across components. The Composition API allows developers to encapsulate component logic into composable functions, improving readability and scalability.
Key Features
- Logic Composition: Enables reuse of logic across multiple components.
- Scalability: Enhances maintainability in large projects.
- TypeScript Friendly: Works seamlessly with TypeScript.
Example: Traditional Options API vs Composition API
// Options API
export default {
data() {
return {
count: 0
};
},
methods: {
increment() {
this.count++;
}
}
};
// Composition API
import { ref } from "vue";
export default {
setup() {
const count = ref(0);
const increment = () => count.value++;
return { count, increment };
}
};
2. Creating Components with the setup()
Function
The setup()
function is the entry point of the Composition API. It serves as a replacement for the data
, methods
, and computed
options in the Options API, enabling a cleaner way to manage component logic.
How It Works
setup()
is called before the component is created.- The function receives two arguments:
- Props: The properties passed to the component.
- Context: Provides access to attributes like slots and emit events.
Example: Basic Component
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { ref } from "vue";
export default {
setup() {
const count = ref(0);
const increment = () => count.value++;
return { count, increment };
}
};
</script>
Benefits
- Improved code organization.
- Simplified logic sharing across components.
3. Reactive References Using ref()
and reactive()
Vue 3’s Composition API introduces two primary ways to handle reactivity: ref()
and reactive()
.
3.1. Using ref()
The ref()
function creates a reactive reference for primitive values, enabling reactivity by wrapping values in a reactive object.
Example:
import { ref } from "vue";
const count = ref(0);
console.log(count.value); // Access using `.value`
count.value++;
console.log(count.value); // Updated value
Use Cases:
- Reactive primitive values like numbers, strings, or booleans.
- Simplifies managing individual values.
3.2. Using reactive()
The reactive()
function creates a reactive object or array. It is ideal for managing complex states.
Example:
import { reactive } from "vue";
const state = reactive({
name: "John",
age: 30,
});
state.age++;
console.log(state.age); // 31
Use Cases:
- Reactive objects or arrays.
- Useful for managing deeply nested states.
3.3. Key Differences Between ref()
and reactive()
Feature | ref() | reactive() |
---|---|---|
Use Case | Primitive values | Objects and arrays |
Access | .value for values | Direct property access |
Reactivity Depth | Shallow | Deep |
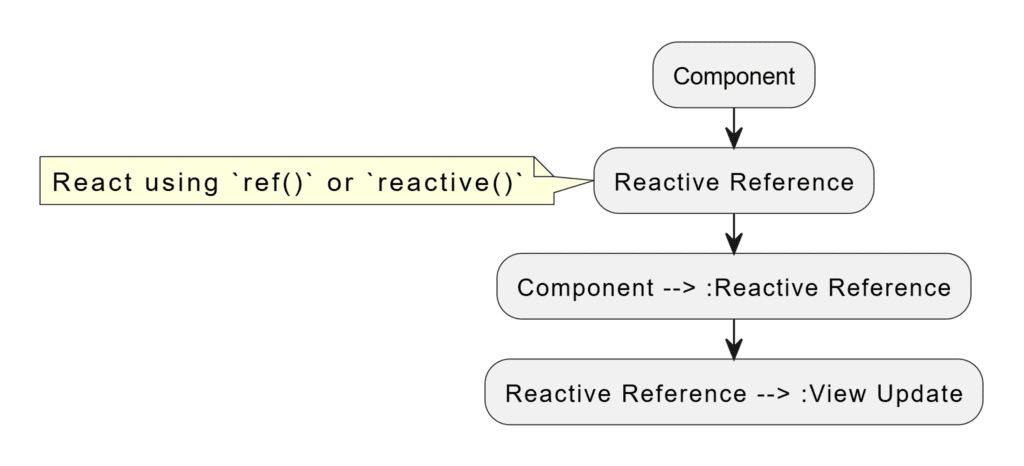
Top 10 Interview Questions on Vue 3 Composition API with Answers
1. What is the Vue 3 Composition API, and why is it introduced?
Answer: The Composition API is a function-based approach to organizing component logic in Vue 3. It was introduced to overcome limitations in the Options API, such as difficulties in reusing and organizing logic across components.
2. How does the setup()
function work in Vue 3?
Answer: The setup()
function is called before a component is created and is used to define its logic. It receives two arguments:
- Props: Contains the props passed to the component.
- Context: Provides access to attributes like
attrs
,slots
, andemit
.
3. What is the difference between ref()
and reactive()
?
Answer:
ref()
: Used for primitive values; reactivity is accessed using.value
.reactive()
: Used for objects and arrays; reactivity is accessed directly.
4. How can you use computed properties in the Composition API?
Answer: You can use the computed()
function to create reactive, computed properties.
import { ref, computed } from "vue";
const count = ref(0);
const doubled = computed(() => count.value * 2);
5. What are the lifecycle hooks available in the Composition API?
Answer: The Composition API provides lifecycle hooks like:
onMounted()
: Called when the component is mounted.onUpdated()
: Called when the component updates.onUnmounted()
: Called when the component is destroyed.
6. How do you share logic between components using the Composition API?
Answer: Logic can be shared using composable functions, which are plain JavaScript functions that encapsulate reusable logic.
Example:
export function useCounter() {
const count = ref(0);
const increment = () => count.value++;
return { count, increment };
}
7. Can you use ref()
for reactive objects?
Answer: Yes, but it is more common to use reactive()
for objects. Using ref()
with an object will still wrap it in a reactive proxy, but you’ll need to use .value
to access or update it.
8. How does the Composition API handle TypeScript?
Answer: The Composition API integrates seamlessly with TypeScript. Types can be defined for props, returned objects, or arguments passed to composable functions.
9. What are the benefits of using the Composition API for large-scale projects?
Answer:
- Improved code reusability through composable functions.
- Cleaner and more maintainable structure.
- Enhanced testability and scalability.
10. How does Vue handle reactivity in nested objects with the Composition API?
Answer: Vue uses a proxy-based reactivity system. When you use reactive()
, it tracks changes deeply in nested objects. For ref()
, you’ll need to handle nested reactivity manually.
The Vue 3 Composition API is a game-changer for modern web development, offering enhanced reusability, scalability, and readability. With its powerful features like ref()
, reactive()
, and the setup()
function, developers can build complex applications with ease.